Python Rock-Paper-Scissors Game Project
- Bilal Khan
- May 25, 2021
- 3 min read
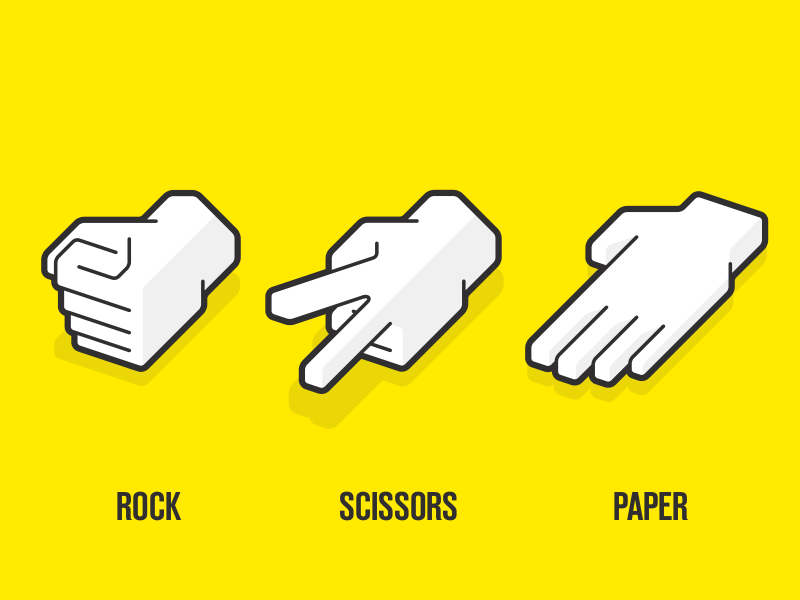
Rock paper scissors is a hand game usually played between two people, in which each player simultaneously forms one of three shapes with an outstretched hand. The object of the rock-paper-scissor python project is to build a game for a single player that plays with a computer, anywhere, and anytime. This project is base on the rules that:
rock blunts scissors so rock wins
scissors cut the paper so scissors win
paper cover rock so paper wins
This project is build using tkinter, random modules, and the basic concept of python.
In this python project, players have to choose any one from rock, paper, and scissors. Then click on the play button will show the result of the game. To implement this python rock paper scissors project we will use the basic concept of python with tkinter and random module.
Tkinter is a standard GUI library which is one of the easiest ways to build a GUI application.
random module use to generate random numbers
To install the libraries we can use the pip installer command on the command prompt: pip install tkinter pip install random Download Code of Rock-Paper-Scissors Python Project
Please download the source code of rock paper scissors project: https://github.com/khnbilal/Python-Rock-Paper-Scissors-Game 1. Importing Libraries:
The first step is to import libraries. Here, we required two modules so we need to import Tkinter and random modules.
2. Initialize Window: Tk() use to initialized Tkinter to create window
geometry() sets the window width and height
resizable(0,0) by this command we can fix the size of the window
title() used to set the title of the window
bg = ‘’ use to set the colour of the background Label() widget used when we want to display text that users can’t modify.
root is the name of our window
text which displays on the label as the title of that label
font in which form the text is written
pack used to the organised widget in form of block (THESE ARE THE APPLICATIONS OF THE FUNCTIONS USED IN THE CODE) 3. For User Choice:
user_take is a string type variable that stores the choice that the user enters.
Entry() widget used when we want to create an input text field.
textvariable used to retrieve the text to entry widget
place() – place widgets at specific position
4. For Computer Choice: random.randint() function will randomly take any number from the given number.
Here we give the if-else() condition to play rock paper scissors
If the computer choose 1 then the rock will set to comp_pick variable
If the computer choose 2 then the paper will set to comp_pick variable
If the computer choose 3 then scissors will set to comp_pick variable
5. Function to Start Game: (Code is given in the above link) 6. Function to Reset:
user_take is a string type variable that stores the choice that the user enters.
We give if-else() condition to check who wins between user choice and computer choice.
In this rock paper scissors game, a player who chooses rock will win by another player who chooses scissors but loose by the player who chooses paper; a player with paper will loose by the player with the scissors. If both choose the same then the game will tie. (Code is given in the above link)
7. Function to Exit:
This function set all variables to an empty string.
root.destroy() will quit the rock paper scissors program by stopping the mainloop().
8. Define Buttons:
Button() widget used when we want to display a button.
command called the specific function when the button will be clicked.
root.mainloop() method executes when we run our program.
*PROGRAM OUTPUT*
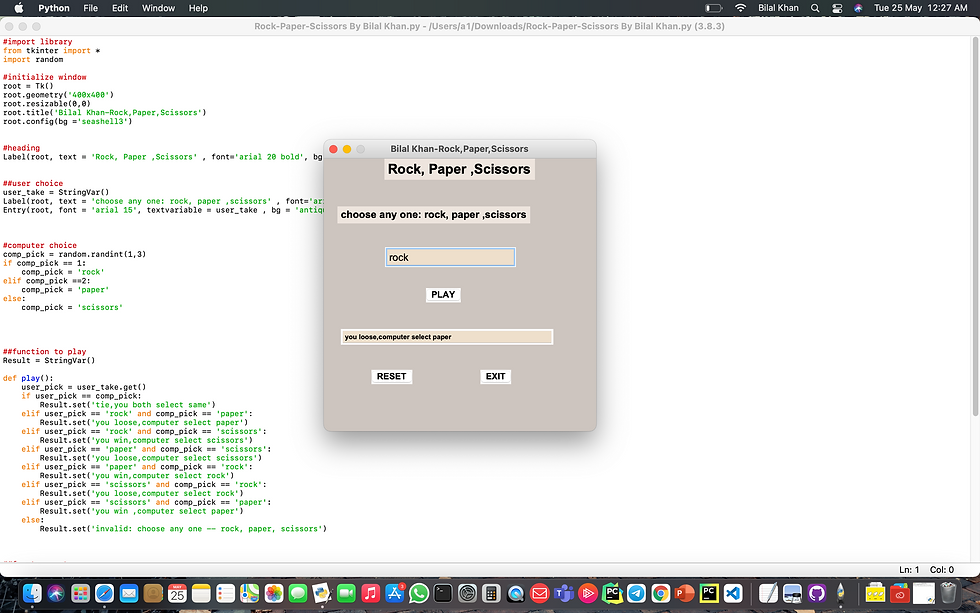
Comments